In INOSIM simulations, custom stochastic unit failures can be utilized to accurately replicate reality in a plant. With the Statistical Analysis Add-on, a large number of simulations can be conducted and then be stochastically evaluated. In a previous Tip & Trick titled Two Ways Of Simulating Unit Failures, the default usage of unit failures in INOSIM was already described. This tip & trick focuses on simulating custom behavior triggered by a failure. Two application examples are provided here.
Example 1: Cancelling Orders in Case of a Failure
Application of Failure Control and the Cancel Stage
Before we delve into the first example, let’s take a look at the different stages in an INOSIM recipe. There are four different stages: Setup, Normal, Cleaning, Cancel (Figure 1).

The Setup stage is always executed first, followed by the Normal stage. Finally, the Cleaning stage is executed. The Setup stage is typically only executed for the first batch of an order, while the Cleaning stage is only executed at the end of the last batch. The Cancellation stage represents a special case, as it is only called when an order is cancelled using the cancel method in VBA.
More information on this can be found in the documentation under Recipes and Recipe Modules > Execution of master recipes > Execution of unit operations > Stages.
By default, operations are inserted as normal stage. To change the stage, right-click on an operation, and then you can select the corresponding stage (Figure 2).
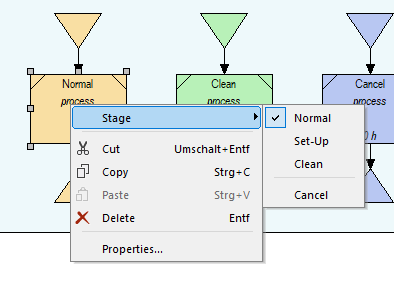
In the first example, we have a look at a batch reactor with a simple recipe (Figure 3). Initially, reactants are transferred into the reactor, followed by the reaction and sampling. Finally, the product is filled into a product container. Subsequently, in the Cleaning stage (green), the reactor is cleaned. In the event of a failure, the order should be cancelled, the remaining material in the tank be discarded, and the reactor be cleaned. Since these steps should only be executed in the event of a Cancellation, they are part of the Cancellation stage (blue).

The failures on the reactor are defined here via the user interface (Figure 4). The interval of the failure is determined by a normal distribution with a mean of two days, a standard deviation of twelve hours, and a lower bound of zero. The duration is described by a uniform distribution between zero and five hours.

In normal circumstances, the operations active on a unit during a failure are only extended by the duration of the failure; the order is not cancelled. To cancel the order at the beginning of the failure and trigger the Cancellation phase, a failure control can be used. A failure control is always called at the beginning and end of a failure. The failure is entered in the unit settings under the Failures tab, as shown in Figure 4. The control for our example looks like this:
Sub Reactor_Failure(u As Unit, reason As FailureControlArg)
Dim o As Order
If reason = FailureControlArg.bFailureBegin Then 'When the Failure begins
For Each o In u.ActiveOrders 'All Active Orders on Failed Unit are cancelled
o.Cancel 'Cancel-Method for the order
Next
End If
End Sub
The two predefined variables in this control are u as Unit and reason as FailureControlArg, where u represents the unit from which this control is called, and reason indicates whether the control is called at the beginning or end of the failure. In the control, it is first checked whether it is the beginning of the failure, and then all active orders on the unit are looped through and cancelled. This cancellation then triggers the Cancel stage. In Figure 5, you can see the Gantt chart for the described process, colored according to the stages. A failure occurs on January 5th (highlighted in red). Following the failure, the Cancel stage is executed (orange). In the level graph, you can also see that the unit is emptied. Then, a cleaning step is performed (Clean Fail).
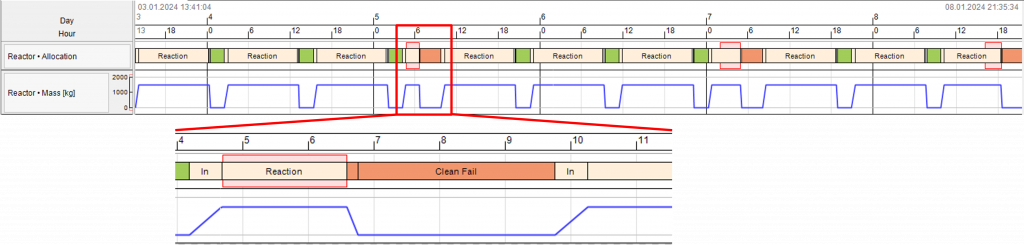
A failure control cannot only be used to cancel an order, like we did it in this example. Further possible applications could be: Restart an order in case of a failure or activate an alternative unit, where the production can go on.
Example 2: Continuous Production – Failure Leads to Off-Spec Product
In the second example, we look at continuous production. The recipe consists only of continuous transfer in the normal stage followed by a Cleaning phase (s. Figure 6).

In this example, a failure should result in the production of an off-spec product. The plant is not stopped, and the transfer is not halted either. In this case, the recipe depicted in Figure 7 is executed. Here, instead of transferring the material into the product tank, it is transferred into an additional tank for the off-spec material.
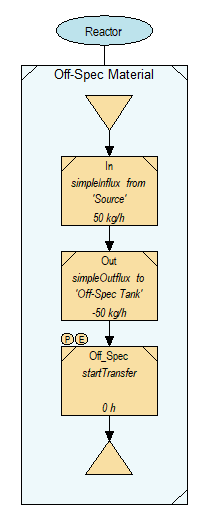
Since production should not be interrupted here, working with a traditional failure is not feasible, as it always causes the current step on the disrupted unit to pause. Therefore, in this example, the failure is created manually through a workaround in VBA. To do this, the two distributions used in the first example for the duration and interval of the failure are created first in the initialization: a uniform distribution for the duration and a normal distribution for the frequency.
'Initialize Off-Spec Production
norm = New Normal 'Normal Distribution
uni = New Uniform 'Uniform Distribution
'Interval of Failures
norm.Mu = 2*24*3600 ' Mean Value: 2d in seconds
norm.Sigma = 12*3600 'Standard deviation: 12h in seconds
norm.LowerBound = 0
'Duration of Failures
uni.Start = 1*3600 'Lower Bound: 1h in seconds
uni.Stop = 5*3600 'Upper Bound: 5h in seconds
Additionally, a subroutine has been created that generates an order for the off-spec material and cancels the current order on the unit which is also used as input for the routine (u as Unit).
Sub OffSpec_Production ( u As Unit)
Dim o As Order
count_OffSpec += 1 'counter to create unique order names
'create new order that produces Off-Spec Material
o = New Order
o.Recipe =Recipes ("Off-Spec Material (V1)")
o.Name = "Off-Spec Material " & count_OffSpec
Orders.Add o
'Cancel all other active orders on the unit
For Each o In u.ActiveOrders
o.Cancel
Next
End Sub
Next, you need to use the distributions to call the described subroutine at the right points in time. To do this, we use the ScheduleSub method. The method is used to call subroutines at specified timepoints, independent of events in the simulation. As input, the method first requires the name of the subroutine as a string, then a time or date value, and an indication of whether it is a relative or absolute time to determine the timing of the call. Lastly, optional arguments for the subroutine can be passed (see Figure 8).

In our example, the ScheduleSub Method will be called like this:
Simulation.ScheduleSub("Macro1.OffSpec_Production",norm.Sample,DateReferenceType.bRelative,Units("Reactor"))
In this example, the timing of the call is specified as a relative value from the current simulation time. This time is a value from our normal distribution for the interval of occurrence of failures. Additionally, the reactor is passed as the unit on which the failure should occur for the subroutine.
The duration of the failure is then set in a parameter control in the recipe for the off-spec material for the Off-Spec operation. To do this, a value from the distribution for the duration of the failure is used.
Sub Off_Spec_Duration(cop As OrderOperation, mop As RecipeOperation)
'duration is a sample form the uniform distribution
cop.Duration = uni.Sample
End Sub
As a final step, at the end of the failure, a new order for the production of the standard product is initiated, and the next failure is scheduled using the ScheduleSub method.
Sub Restart_Production(cop As OrderOperation)
'at the end of the Off-Spec Production a new normal production order is started
count+=1
Dim o As New Order
o.Name = "Production Order " & count
o.Recipe = Recipes("Continuous Production (V1)")
Orders.Add o
'to call next Off-Spec production ScheduleSub is used again, using a sample form the normal distribution representing the inteval of failure
Simulation.ScheduleSub ("Macro1.OffSpec_Production",norm.Sample,DateReferenceType.bRelative,Units("Reactor"))
End Sub
In the resulting Gantt chart from this example (Figure 9), you can now clearly see the periods during which off-spec material is produced (green). In the level graph, it’s also evident that during these periods, the material is transferred into the Off-Spec Tank, while otherwise the Product Tank is being filled.

In the Download area, the model example can be downloaded, together with a PDF outprint of this Tip & Trick.
More Questions?
Want to know more about this topic or have another question? Please contact us!
More Tips & Tricks
Transfer Analysis with Power BI
In this tip and trick, the Transfer Analysis dashboard is introduced. It is an extension of the Power BI standard dashboard that allows you to…
Animated Graphical Objects In INOSIM
Graphical auxiliary functions give live to your plant layout: By animation, you dynamically display filling level changes, transport movements of objects, or the status of…
Preventing Runtime Errors and Custom Error Handling
This Tip & Trick describes a multitude of methods to prevent and handle runtime errors of your VBA code. You will be introduced to the…